Creating a Camera Kit Web application with React & TypeScript
Camera Kit Web brings Snap's cutting-edge augmented reality technology to your mobile and desktop web applications.
In this tutorial, you'll learn how to build a Camera Kit Web application with React and TypeScript, allowing you to apply a Lens to your webcam.
In subsequent tutorials, we'll enhance the application by adding features like Lens selection, camera switching (if multiple cameras are available), photo capture, and video recording.
Prerequisites
- Before we get started, it's important you familiarize yourself with the core prerequisites of building a web application.
- Node.js and npm must be installed on your computer. npm comes bundled with Node.js by default and can be downloaded here.
- Familiarity with HTML & CSS.
- Basic knowledge of programming; in particular JavaScript (or even better, TypeScript).
- General understanding of React.
- For writing code, we will be using Visual Studio Code (VS Code).
Using VS Code, open the starting project which can be downloaded in the tutorial summary above.
Use the following command to install node modules:npm install
Install Camera Kit Web:npm i @snap/camera-kit
Now, let's start the local dev server:npm run dev
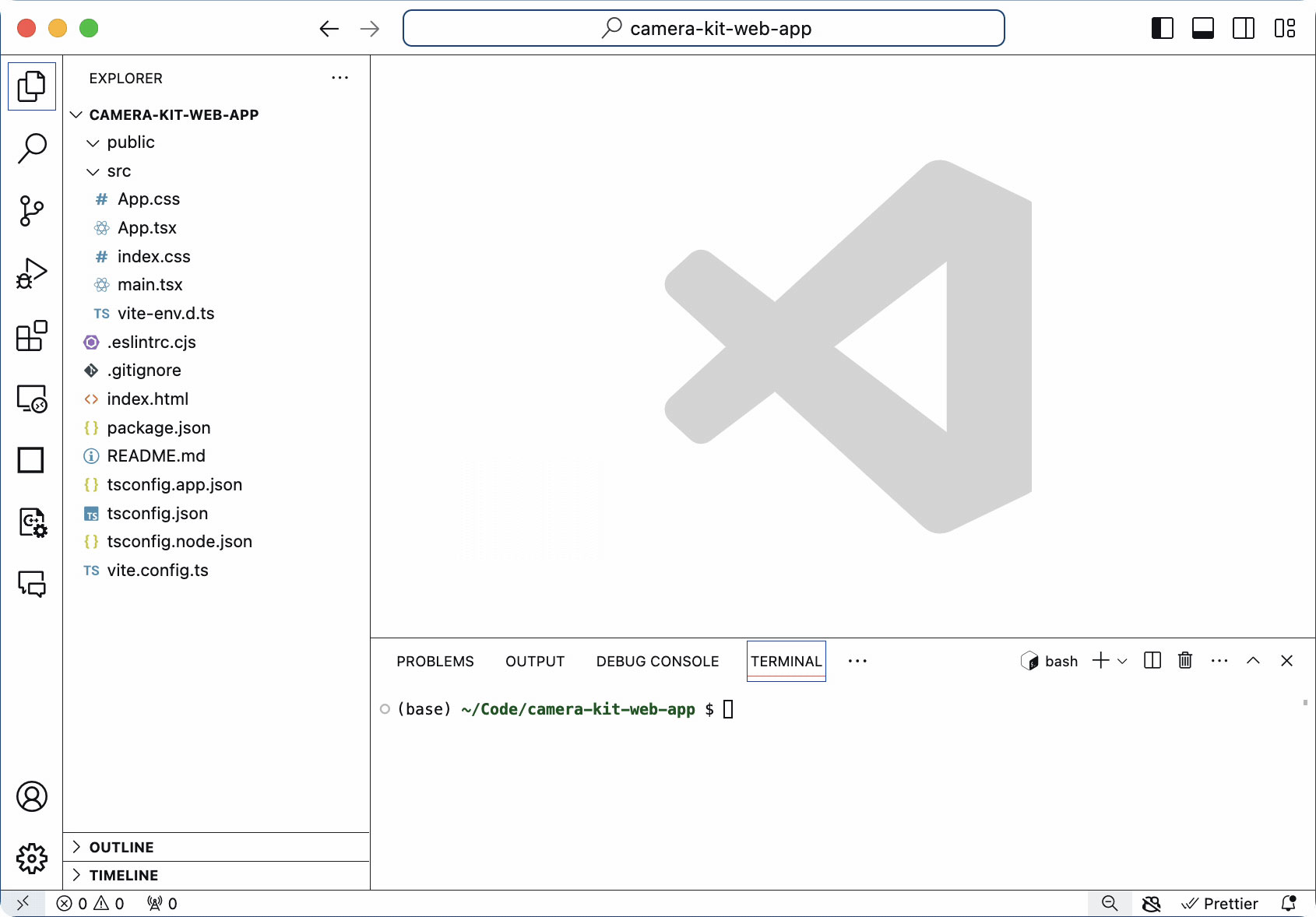
Create a Camera Kit context
The CameraKitContext
will encapsulate all the logic necessary to manage our Camera Kit session. This will ensure that Camera Kit is initialized only once but remains available for use throughout your application.
In the Explorer view, create a new file in the src/contexts
directory named CameraKitContext.tsx
Import necessary modules
Import the necessary modules from the @snap/camera-kit
and React
packages.
Define API token and Lens group ID
These can be found in My Lenses and will be used to bootstrap Camera Kit as well as fetch Lenses.
Define CameraKitState interface
This represents the properties available to components within this context.
Create the context
Initially, set the context value to null
. After Camera Kit is initialized, the context will be populated with the appropriate data.
Create a CameraKit
wrapper component
This component can be used to wrap parts of the application where Camera Kit will be used.
Create a reference to track initialization
To ensure Camera Kit is only bootstrapped once, create a reference with useRef
to be used to track when Camera Kit has been initialized.
Define state for the Camera Kit session and Lenses
These values will ultimately become accessible by consumers of the CameraKitContext
.
Create a Effect that calls a new initializeCameraKit
function
This Effect will set all the logic to initialize the Camera Kit Sesson and fetch Lenses.
Bootstrap Camera Kit
Using the API token defined at the top of this file, bootstrap Camera Kit.
Create a Camera Kit Session
This session will be used to manage video sources and apply Lenses.
Fetch Lenses
Using the Lens Group ID defined at the top of this file, fetch Lenses from the Camera Kit backend.
Set the Camera Kit Session and Lenses to state.
Implement the isInitialized
reference
To prevent Camera Kit from being initialized multiple times, check if isInitialized
is true and return early if it is; otherwise, set isInitialized
to true.
Render a loading state
While Camera Kit is initializing and there is no active session, render a loading state.
Provide the Camera Kit context
Once Camera Kit is initialized and we have an active session, provide the context to the children components.
The children components will have access to both the Camera Kit session and the Lenses from the specified Lens group.
Create a hook for the Camera Kit context
To make the Camera Kit context easily accessible in multiple components and ensure it's only used within its provider, create a custom hook to encapsulate the logic.
Create a file in the src/hooks
directory named useCameraKit.ts
Import necessary modules
Import the useContext
method from React
along with the recently created CameraKitContext
.
Access the CameraKitContext
value
This value contains the Camera Kit session and array of Lenses.
Add a guard clause
Ensure that the hook is used within a component that is wrapped by the CameraKitContext
provider. If the context value is null, throw an error.
Using Camera Kit to apply Lenses
With the Camera Kit context and custom hook in place, it's time to attach a webcam as a source, apply a Lens, and render the output canvas.
Provide the Camera Kit context
In main.tsx
, use the CameraKit
component to provide the context to the App
component and its children. This ensures that all components within App
can access the Camera Kit context.
Using the Camera Kit context
Open App.tsx
and import the necessary modules. This is the component where the Camera Kit session and Lenses will be used.
Access Camera Kit State
Inside the App
component, use the useCameraKit
hook to access the Camera Kit session and Lenses.
Create a reference to the HTMLDivElement
where Camera Kit will render
Use the useRef
React hook to create a reference called canvasContainerRef
and attach it to the returned HTMLDivElement
.
Create a callback function to initialize Camera Kit and invoke it within an effect
This function will contain all the logic to obtain a MediaStream from the webcam and apply a Lens.
Obtain a MediaStream
from the webcam
Additional options like resolution, frame rate and facing mode can be set here as well.
Create a media stream source
Using the createMediaStreamSource
method from the @snap/camera-kit
package, create a media stream source and attach it to your Camera Kit session.
This will also mirror the input feed by utilizing the transform
option.
Apply a Lens
Using the lenses
object from the custom hook, apply the first Lens to the Camera Kit session.
Play the live output canvas
This will immediately start rendering frames from the webcam, with the Lens applied, to the output canvas.
Render the output canvas
Inside of an effect, replace the canvasContainerRef
element with the live output canvas from the Camera Kit session.