Inclusive Camera
Overview
Snap's Inclusive Camera allows users to be seen the way that they want to be seen. Features like Ring Light and Tone Mapping work to improve low light flash capture and exposure, so our camera can start to account for all skin tones and undertones.
Ring Light
The Ring Light feature allows users to see their face in low light conditions and improves front-facing flash by enabling color temperature selection to best match skin undertones. When Ring Light is enabled on the front-facing camera, a soft illuminated border appears around the preview screen to light the user's face and function as a pre-capture flash. The gradient intensity and color can be controlled in the Flash widget.
By default, a Regular Flash is used instead of the gradient Ring Light. For details on how to use Ring Light in your Camera Kit application, see the Android implementation examples below.
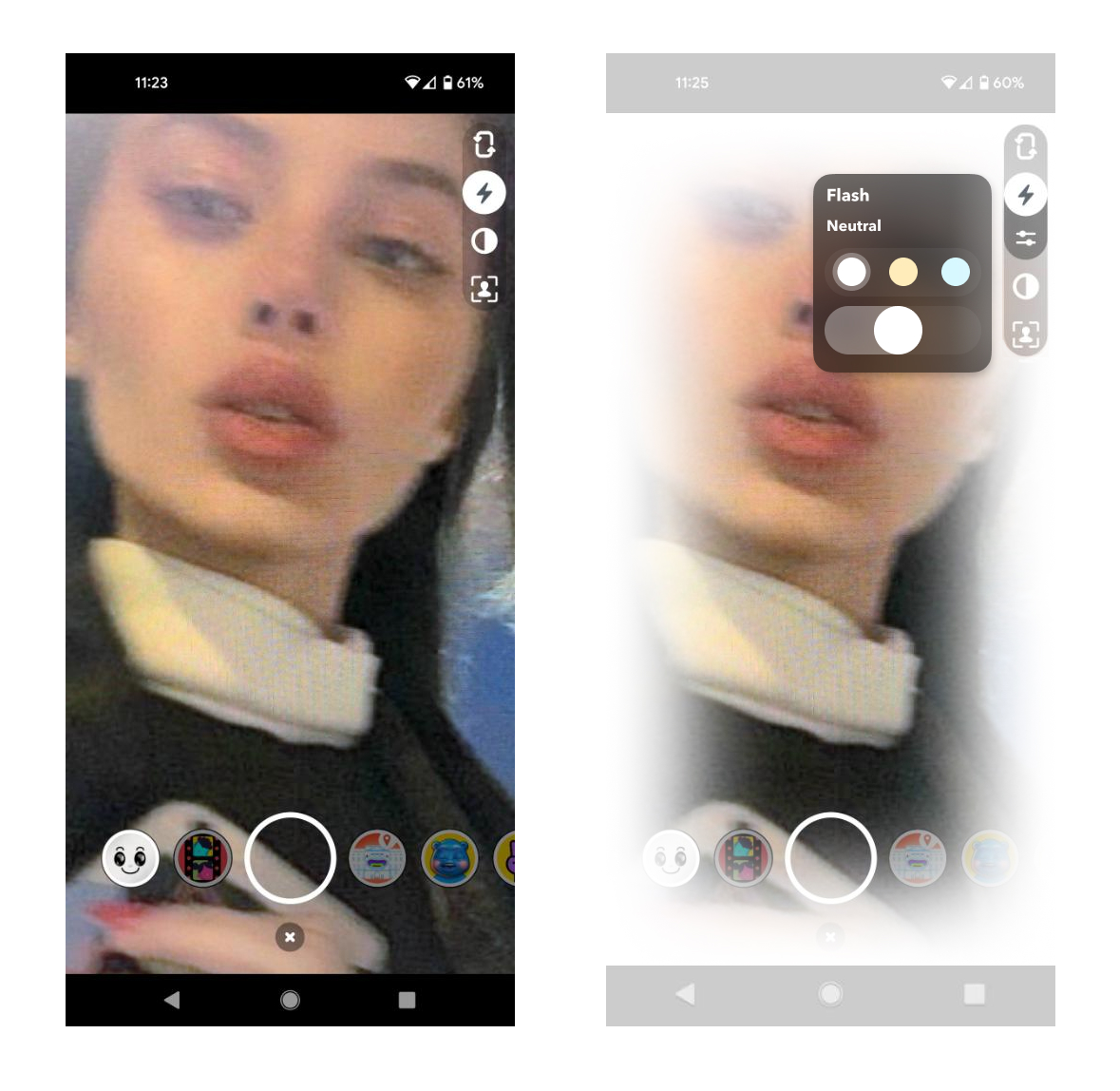
Tone Mapping
Tone Mode is designed to fine-tune light exposure and better account for all skin tones, by selectively modifying areas that show up too dark or too bright. For example, it can brighten shadows and reduce overly light areas.
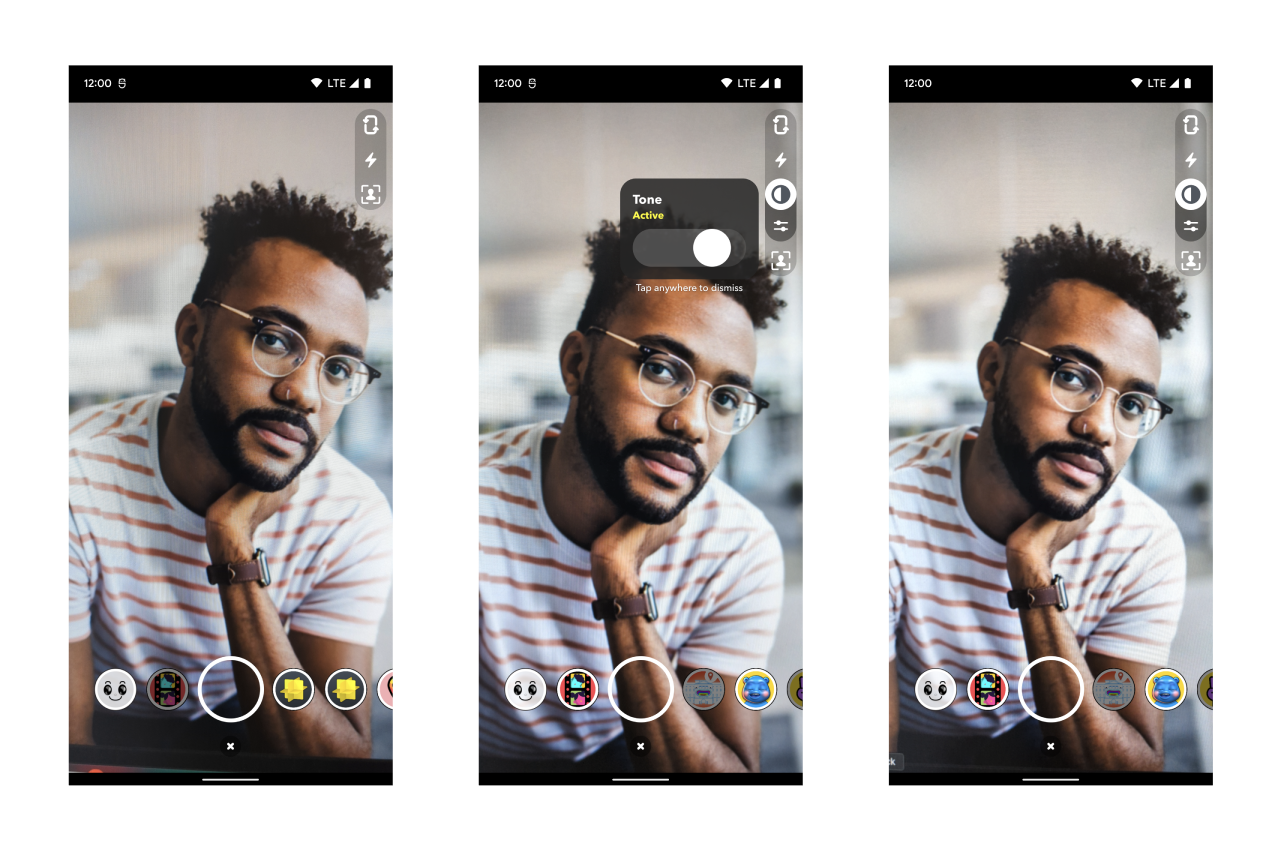
Sample Apps
Sample apps that demonstrate how to implement Inclusive Camera features are available for you to reference and download on GitHub.
Android Implementation
To implement Inclusive Camera features in your Android application, use the Android Camera Kit SDK. Before you implement any features, please determine whether you are using CameraLayout
and check if the feature is available on your device.
CameraLayout Usage:
-
If you are using CameraLayout, it provides a MutableSet of
AdjustmentsComponent.Adjustments
withAdaptiveToneMappingAdjustment
for Tone Mapping. These adjustments are enabled by default on CameraLayout, but you can disable them with:cameraLayout.enabledAdjustments = emptySet()
-
If you are not using CameraLayout, you can apply these adjustments directly on the session object as shown in the following examples.
Check Feature Availability:
-
Some devices may not support these features, so you should first check if the feature is available on the device before proceeding with implementation. For example, you can check if
AdaptiveToneMappingAdjustment
is available:session.adjustments.processor.available(AdaptiveToneMappingAdjustment) { available ->
if(available) {
// apply the adjustment
}
}
Android Ring Light
Ring Light illuminates the boundaries of the screen when the front-facing camera is on. Users can select the color and intensity of the light.
If you are using CameraLayout, you can toggle ring light on or off by setting the boolean value to cameraLayout.flashBehavior.shouldUseRingFlash
.
Ring Light is offered via a support package support-snap-flash
and can be configured through the FlashBehavior.kt
class, which is also where the Ring Light control widget is constructed and connected to the Ring Light view and flash toggle view. You can find the view’s implementation in the RingFlashView.kt
file in the Camera Kit support package.
If you are not using CameraLayout, then you can use the support package that provides RingFlashView.kt
for ring light, SolidFlashView.kt
for solid front facing flash, FlashToggleLayout.kt
for a flash toggle that also controls the visibility of the ring light widget, and FlashBehavior.kt
to connect and configure all these pieces together. To set it up:
val flashBehavior = FlashBehavior(
context,
<FlashToggleLayout view>,
<SolidFlashView that is inflated in your own view hierarchy above other views>,
<CameraControlMenuLayout used as ring light control widget>,
<Widget style>
)
flashBehavior.shouldUseRingFlash = true
This will turn on Ring Light if flash is toggled on. You must also do the following to have the Ring Light be inflated behind the carousel as to not cover it when active:
configureCarousel {
attachViewTo(LensesComponent.Carousel.LayoutPlacement.Behind) { viewStub ->
// Adding closeable to be closed when root view detached
flashBehavior.attachRingFlash(viewStub).addTo(closeOnDetach)
}
Android Tone Mapping
AdaptiveToneMappingAdjustment
provides an image processing algorithm that remaps image color space to better highlight a person's face based on its skin tone. You can adjust the tone amount (float range from 0 to 1):
session.adjustments.processor.apply(AdaptiveToneMappingAdjustment) { result ->
result.whenApplied { applied -> applied.controller.amount = 0.75f }
}