Motion Controller Module
Overview
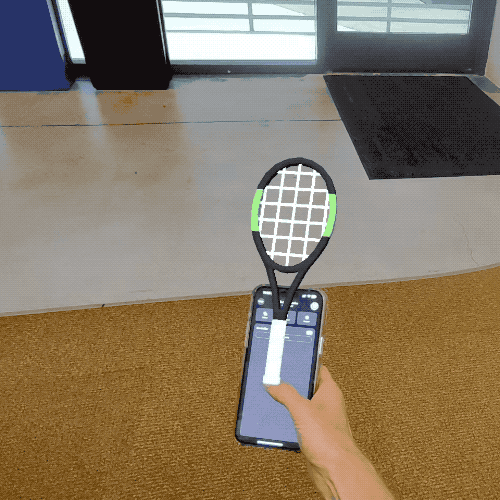
Developers for Spectacles are able to build mobile controllers that are able to communicate to Spectacles that are linked to the mobile companion app Spectacles App. The following guide explains how to integrate a motion controller into a Lens Studio project and utilize its api.
This Motion Controller Module is designed to support a variety of 3rd party input devices in future. At the moment only the Spectacles App controller is supported and only one Motion Controller can be connected to Spectacles at a time. In the long future, we expect that there may be multiple Motion Controllers simultaneously.
Motion Controller allows access to track world position and rotation of a mobile controller, track touch events and invoke haptic feedback on the mobile device.
Example
Let’s build a simple example that would allow us to manipulate a 3D object in space and provide certain interactions.
Prerequisites
Please configure your Lens Studio project for Spectacles and connect your Spectacles device.
Also please have the Spectacles App installed on your phone.
Adding a Motion Controller to Your Project
You can access the Motion Controller through the Motion Controller Module asset like this:
//@input Asset.MotionControllerModule motionControllerModule
const motionController = script.motionControllerModule.getController();
Alternatively, you can import it as a module using the require
keyword:
const MotionControllerModule = require("LensStudio:MotionControllerModule");
const motionController = MotionControllerModule.getController();
Simple Transform Controller
Create a new scene object and a new child object of your choice. This is the 3D object we’ll be manipulating.

Scale and move the child object as shown below.
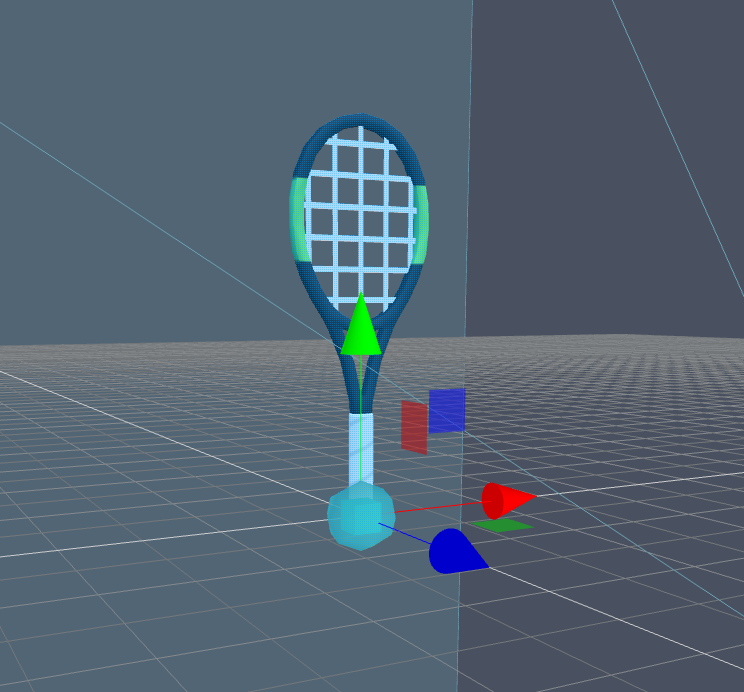
Then create a new TypeScript file in Asset Browser and paste the code:
- TypeScript
- JavaScript
const MotionControllerModule = require('LensStudio:MotionControllerModule');
@component
export class NewScript extends BaseScriptComponent {
private transform;
private controller;
onAwake() {
var options = MotionController.Options.create();
options.motionType = MotionController.MotionType.SixDoF;
this.controller = MotionControllerModule.getController(options);
this.transform = this.sceneObject.getTransform();
this.controller.onTransformEvent.add(this.updateTransform.bind(this));
}
updateTransform(position, rotation) {
this.transform.setWorldPosition(position);
this.transform.setWorldRotation(rotation);
}
}
const MotionControllerModule = require('LensStudio:MotionControllerModule');
let options = MotionController.Options.create();
options.motionType = MotionController.MotionType.SixDoF;
const motionController = MotionControllerModule.getController(options);
const sceneObject = script.getSceneObject();
const transform = sceneObject.getTransform();
motionController.onTransformEvent.add((worldPosition, worldRotation) => {
transform.setWorldPosition(worldPosition);
transform.setWorldRotation(worldRotation);
});
Save the script and add it to the parent scene object we created above.
Just like this the simplest example is ready. Click on the `Preview Lens` button and select `Send to Connected Spectacles`. Open the Spectacles App, go through the calibration step and enable the `Controller` toggle.

At this point you should see the object move and rotate with your phone!
You may find the complete Motion Controller Helper example in Lens Studio Asset Library!
Using Touch Events
Let’s expand our code by adding a couple lines :
- Add another script input:
@input
childObject: SceneObject
- Add an `onTouchEvent` function:
onTouchEvent(normalizedPosition, touchId, timestampMs, phase) {
if (phase != MotionController.TouchPhase.Began) {
return
}
this.childObject.enabled = !this.childObject.enabled;
}
- And finally add an event callback in onAwake function
this.controller.onTouchEvent.add(this.onTouchEvent.bind(this))
Send the lens to Spectacles and try tapping on the screen to toggle the object.
The Motion Controller also invokes usual script touch events such as TapEvent, TouchStartEvent, TouchMoveEvent and TouchEndEvent. Which means you can reutilize some of existing helper scripts and create touch interactions that also work in Lens Studio.
Adding Haptic Feedback
Motion Controller allows you to invoke the tactile response or physical sensations provided by a device when a user interacts with it.
For this api please make sure you have Vibration and System Haptics enabled on your phone.
Let’s expand an `onTouchEvent` function by adding vibration feedback whenever a 3D object is enabled:
onTouchEvent(normalizedPosition, touchId, timestampMs, phase) {
if (phase != MotionController.TouchPhase.Began) {
return
}
this.childObject.enabled = !this.childObject.enabled;
if (this.childObject.enabled) {
var request = MotionController.HapticRequest.create()
request.hapticFeedback \= MotionController.HapticFeedback.VibrationMedium
request.duration = 1.0
this.controller.invokeHaptic(request)
}
}
Please check out the full Motion Controller API documentation for more code snippets and detailed functionality description!