Leaderboard
Spectacles offers APIs to create Leaderboards so users of your Lens can track their progress and compare scores with friends and users worldwide.
Privacy Note: Leaderboards protect user privacy and require each user to opt in to share scores.
For Spectacles we only support the Leaderboard Module, not the Leaderboard Component that's used by mobile Snapchat. The Leaderboard Module provides access to raw Leaderboard data, but unlike the Leaderboard Component it does not provide any UI.
Usage
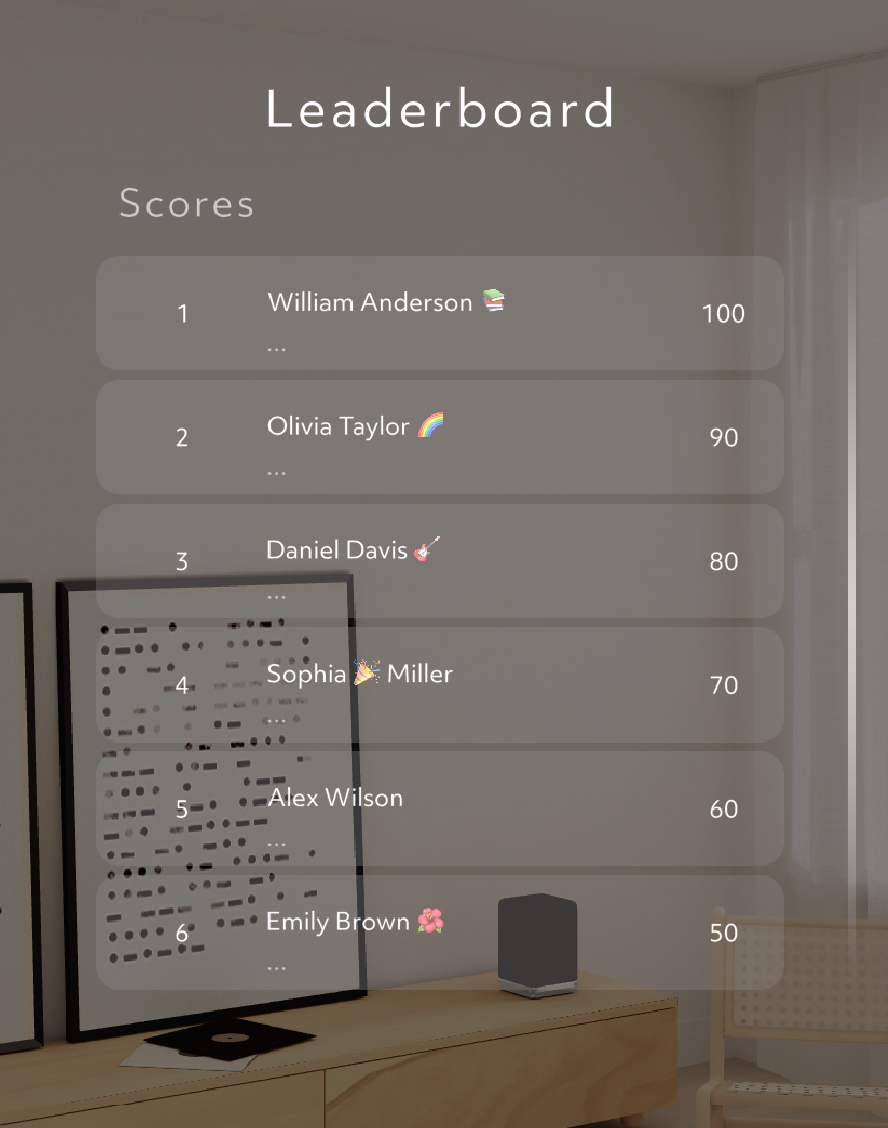
Prerequisites
- Lens Studio v5.7.0 or later
- Spectacles OS v5.60.x or later
Creating Leaderboards
To create a new Leaderboard add the LeaderboardModule to your project and include it in your scripts. The LeaderboardModule enables you to create or retrieve a Leaderboard through the getLeaderboard method, which takes a CreateOptions parameter where you can set:
- name: The name of the Leaderboard. Your Leaderboard is uniquely determined by the combination of your Lens ID and this leaderboard name. If a Leaderboard with this name does not exist, it will be created for this lens. If the Leaderboard with this name does exist, it will simply be returned.
- orderingType: Ascending or Descending.
- ttlSeconds: The number of seconds this leaderboard should exist before being reset.
Once the Leaderboard is created, you can submit scores to it, or retrieve it for display.
Submitting Scores
To submit a score to the leaderboard, simply call the submitScore method, as shown in the example below, where we retrieve a Leaderboard and immediately submit a random score between 1 and 1000.
- TypeScript
- JavaScript
@component
export class LeaderboardExample extends BaseScriptComponent {
private leaderboardModule = require('LensStudio:LeaderboardModule');
onAwake() {
this.updateLeaderboard();
}
updateLeaderboard(): void {
const leaderboardCreateOptions = Leaderboard.CreateOptions.create();
leaderboardCreateOptions.name = 'TEST_NAME';
leaderboardCreateOptions.ttlSeconds = 800000;
leaderboardCreateOptions.orderingType = 1;
this.leaderboardModule.getLeaderboard(
leaderboardCreateOptions,
(leaderboardInstance) => {
let randomInt = MathUtils.randomRange(1, 1000);
leaderboardInstance.submitScore(
randomInt,
this.submitScoreSuccessCallback,
(status) => {
print('[Leaderboard] Submit failed, status: ' + status);
}
);
},
(status) => {
print('[Leaderboard] getLeaderboard failed, status: ' + status);
}
);
}
submitScoreSuccessCallback(currentUserInfo): void {
print('[Leaderboard] submitScore success callback');
if (!isNull(currentUserInfo)) {
print(
`[Leaderboard] Current User info: ${
currentUserInfo.snapchatUser.displayName
? currentUserInfo.snapchatUser.displayName
: ''
} score: ${currentUserInfo.score}`
);
}
}
}
const leaderboardModule = require('LensStudio:LeaderboardModule');
function updateLeaderboard() {
const leaderboardCreateOptions = Leaderboard.CreateOptions.create();
leaderboardCreateOptions.name = 'TEST_NAME';
leaderboardCreateOptions.ttlSeconds = 800000;
leaderboardCreateOptions.orderingType = 1;
leaderboardModule.getLeaderboard(
leaderboardCreateOptions,
(leaderboardInstance) => {
let randomInt = MathUtils.randomRange(1, 1000);
leaderboardInstance.submitScore(
randomInt,
submitScoreSuccessCallback,
(status) => {
print('[Leaderboard] Submit failed, status: ' + status);
}
);
},
(status) => {
print('[Leaderboard] getLeaderboard failed, status: ' + status);
}
);
}
function submitScoreSuccessCallback(currentUserInfo) {
print('[Leaderboard] submitScore success callback');
if (!isNull(currentUserInfo)) {
print(
`[Leaderboard] Current User info: ${
currentUserInfo.snapchatUser.displayName
? currentUserInfo.snapchatUser.displayName
: ''
} score: ${currentUserInfo.score}`
);
}
}
updateLeaderboard();
Displaying Leaderboards
To load a Leaderboard's entries, call getLeaderboardInfo with RetrievalOptions. The RetrievalOptions allow you to specify:
- usersLimit: How many user entries to return.
- usersType: Whether to return the Global Leaderboard or the Friends Leaderboard. The Global Leaderboard returns scores of all users of the Lens, while the Friends Leaderboard only ranks and returns the scores of the current user's friends.
The leaderboard entries are returned in the success callback as an array of UserRecord objects. Below is an example demonstrating how to print the leaderboard entries.
- TypeScript
- JavaScript
@component
export class LeaderBoardInfo extends BaseScriptComponent {
private leaderboardModule = require('LensStudio:LeaderboardModule');
onAwake() {
this.updateLeaderboard();
}
updateLeaderboard(): void {
const leaderboardCreateOptions = Leaderboard.CreateOptions.create();
leaderboardCreateOptions.name = 'TEST_NAME';
leaderboardCreateOptions.ttlSeconds = 800000;
leaderboardCreateOptions.orderingType = 1;
this.leaderboardModule.getLeaderboard(
leaderboardCreateOptions,
(leaderboardInstance) => {
let leaderboardRetrievalOptions = Leaderboard.RetrievalOptions.create();
leaderboardRetrievalOptions.usersLimit = 10;
leaderboardRetrievalOptions.usersType = Leaderboard.UsersType.Global;
leaderboardInstance.getLeaderboardInfo(
leaderboardRetrievalOptions,
(otherRecords, currentUserRecord) => {
print('[Leaderboard] Global scores loaded');
print(
'[Leaderboard] Current user: ' +
currentUserRecord.snapchatUser.displayName +
', Score: ' +
currentUserRecord.score
);
otherRecords.forEach((record) => {
if (
record &&
record.snapchatUser &&
record.snapchatUser.displayName
) {
print(
'[Leaderboard] User: ' +
record.snapchatUser.displayName +
', Score: ' +
record.score
);
}
});
},
(status) => {
print('[Leaderboard] getLeaderboardInfo failed, status: ' + status);
}
);
},
(status) => {
print('[Leaderboard] getLeaderboard failed, status: ' + status);
}
);
}
}
const leaderboardModule = require('LensStudio:LeaderboardModule');
function updateLeaderboard() {
const leaderboardCreateOptions = Leaderboard.CreateOptions.create();
leaderboardCreateOptions.name = 'TEST_NAME';
leaderboardCreateOptions.ttlSeconds = 800000;
leaderboardCreateOptions.orderingType = 1;
leaderboardModule.getLeaderboard(
leaderboardCreateOptions,
(leaderboardInstance) => {
let leaderboardRetrievalOptions = Leaderboard.RetrievalOptions.create();
leaderboardRetrievalOptions.usersLimit = 10;
leaderboardRetrievalOptions.usersType = Leaderboard.UsersType.Global;
leaderboardInstance.getLeaderboardInfo(
leaderboardRetrievalOptions,
(otherRecords, currentUserRecord) => {
print('[Leaderboard] Global scores loaded');
print(
'[Leaderboard] Current user: ' +
currentUserRecord.snapchatUser.displayName +
', Score: ' +
currentUserRecord.score
);
otherRecords.forEach((record) => {
if (
record &&
record.snapchatUser &&
record.snapchatUser.displayName
) {
print(
'[Leaderboard] User: ' +
record.snapchatUser.displayName +
', Score: ' +
record.score
);
}
});
},
(status) => {
print('[Leaderboard] getLeaderboardInfo failed, status: ' + status);
}
);
},
(status) => {
print('[Leaderboard] getLeaderboard failed, status: ' + status);
}
);
}
updateLeaderboard();