Part 2 - Adding Forward Movement, Jump, and Slide
Welcome to Part 2 of the Bitmoji Runner Game tutorial! In Part 1, we laid the groundwork by enabling basic player movement in response to swipe gestures.
We created left and right swipe controls that allowed the Bitmoji to smoothly move side-to-side with animations that matched the direction of the swipes.
In this part of the tutorial, we'll expand the functionality of our Bitmoji by making it move away from us on the z-axis to create the effect of running forward.
We'll also set up the camera to follow the Bitmoji's movement, adding to the dynamic feel of the scene. Additionally, we'll implement the jump animation triggered
by a swipe up and the slide animation triggered by a swipe down.
Prerequisites
- Before we begin, make sure you have Lens Studio (version 5.2 or higher) installed on your computer.
- A basic understanding of programming, especially JavaScript, will be helpful.
- You will also need the project from Part 1 of this tutorial as a starting point.
Before we start building in this part, let's revisit what we accomplished in Part 1.
Here's the final result from Part 1, where the player can move left and right smoothly in response to swipe gestures, with animations dynamically transitioning based on movement.
Now, let's preview what you'll be building in this part.
By the end of Part 2, you'll have a player character that runs forward, with animations for jumping and sliding triggered by swipe gestures.
The camera will also follow the player, adding a dynamic feel to the gameplay.
Making the Player Move Forward
Open the project from Part 1 and start expanding its functionality
Open the project you created in Part 1, as we’ll continue building on it to add new features in this part.
To make the Bitmoji run forward and appear to move away from us, we need to decrease its position along the z-axis.
We'll do this in the onUpdate
function of the PlayerController
script.
First, lets's recall what the currentPos
variable does from Part 1: it stores the current position of the player.
- Initially, the `targetPos` is set to match the `currentPos`.
- Based on user input (e.g., right swipe or left swipe), the `targetPos.x` is updated incrementally in the `onUpdate` function.
- The player’s transform (local position) is then updated every frame to match the `targetPos`, ensuring smooth movement.
At the top of the PlayerController
script, we will define a new variable called speed.
This variable will determine how fast the Bitmoji moves forward.
Set its initial value to 50
, but you can adjust it later to experiment with different movement speeds.
Defining the speed variable allows you to easily control the forward movement without needing to change the logic within the onUpdate
function.
Inside the onUpdate
function, we’ll continuously decrease the currentPos.z value to simulate forward movement.
Multiply the speed variable by getDeltaTime to ensure the movement remains smooth and consistent across different devices.
getDeltaTime adjusts the movement based on the time elapsed between frames, making the Bitmoji's speed consistent regardless of the frame rate.
For example, higher frame rates will process smaller steps, while lower frame rates will process larger steps, ensuring smooth motion.
Finally, update the targetPos.z
to match the updated currentPos.z
value.
This ensures that the player's transform (local position) gets updated correctly each frame, maintaining smooth and synchronized movement.
Now, if you check the Preview panel, you’ll see that the Bitmoji runs away from us.
By adjusting the speed
variable, you can make it move faster or slower.
However, after a few seconds, the Bitmoji will move too far and disappear from view.
To solve this, we need to make the camera follow the Bitmoji, ensuring it stays visible at all times.
Making the Camera Follow the Bitmoji
To ensure the Bitmoji stays visible as it runs forward, we’ll create a script to make the camera follow it smoothly.
Click on the Player scene object. Then, in the Inspector panel, click Add Component and select JavaScript to add a new script component.
This will create a script in the Asset Browser. Click on the newly created script, press Enter to rename it to CameraFollow, and press Enter again to save.
Finally, double-click the CameraFollow script to open it in the script editor.
Giving your script a meaningful name makes it easier to identify its purpose within the project.
Start by declaring the two necessary inputs:
- The target, which will be the Bitmoji.
- The follower, which will be the camera.
These inputs allow us to reference the objects we want to synchronize in the script.
Save the script and go to the Inspector panel.
Assign the follower input to the camera and the target input to the Bitmoji.
This links the script to the objects it will control.
Let's retrieve the Transform components for both the target and follower.
These components allow us to access and manipulate the position of the objects in 3D space.
The getTransform function gives us control over an object’s position
, rotation
, and scale
, which are essential for syncing the follower with the target.
To calculate the initial offset between the follower and the target we need to:
- Retrieve the current world-space position of the
follower
. - Retrieve the current world-space position of the
target
. - Use the
.sub()
method to calculate the vector difference between the two positions by subtracting thetarget
's position from thefollower
's position (x, y, z components).
This ensures that the camera maintains its starting position relative to the Bitmoji as it follows.
Store the initial x position of the follower.
This ensures the camera doesn’t move horizontally and only follows the Bitmoji along the z-axis.
Locking the x position helps keep the camera centered and prevents side-to-side movement.
In the onUpdate function, retrieve the follower's current position in world space using getWorldPosition()
.
This gives us the current location of the follower (the camera) as it moves.
Tracking the follower's current position ensures we can update it accurately to follow the target.
After retrieving the follower’s current position, fix its x position by setting it to the initial follower x value.
This ensures the follower remains aligned horizontally with its initial setup while still following the target’s y (up/down) and z (forward/backward) movements.
By fixing the x position, the camera will follow the target's forward, upward, or downward movements, but its horizontal alignment will remain constant.
Next, calculate the desired position for the follower by adding the offset to the target's current position.
This ensures the follower maintains its relative distance and alignment with the target.
Adding the offset keeps the follower at a consistent distance from the target, creating smooth and natural movement.
Above the onUpdate function, we need to define a constant called followThreshold, which represents the minimum allowable distance between the follower (e.g., the camera) and the target (e.g., the Bitmoji).
If the follower is closer than this distance to the target, it will stop moving.
Setting a small threshold value (e.g., 0.1
) ensures smooth stopping and prevents the follower from jittering when it's close to the target.
Now, back in the onUpdate function, use the distance
method to calculate how far the follower is from the target.
If this distance is greater than the followThreshold, the follower will continue moving closer to the target.
This condition ensures that the follower only moves when it’s far enough from the target, maintaining smooth and controlled behavior.
Next, we need to introduce another constant called smoothFactor below the followThreshold
variable.
This value controls how smoothly the follower
(e.g., the camera) moves towards the target (e.g., the Bitmoji).
A lower value makes the movement smoother and slower, while a higher value makes it faster.
Set smoothFactor to 0.3
initially. This ensures a balance between smoothness and responsiveness. You can adjust this value later based on your gameplay needs.
Back in the onUpdate function, move the follower towards the target position if the distance between them is greater than the follow threshold.
Use vec3.lerp to interpolate between the current position and the target position, creating smooth motion.
vec3.lerp
takes three arguments:
- The follower's current position.
- The target position.
- The smoothFactor.
Bind the onUpdate function to the UpdateEvent
so it runs every frame, keeping the camera synchronized with the Bitmoji’s movement.
By binding the function to the update event, the camera continuously adjusts its position in real-time.
Save the script and test it in the Preview Panel. Notice how the Bitmoji now remains in sight and doesn’t disappear from view.
While it might look like the Bitmoji isn’t running away, it actually is — the camera is following its movement, creating the illusion that the Bitmoji stays at a constant distance.
Test by swiping left and right to see how the camera remains centered while tracking the Bitmoji’s position.
To debug and observe the follower’s behavior, you can print its position in the onUpdate
function by adding: print(followerTransform.getWorldPosition());
.
This will display the follower’s position in the Logger, showing how its z position decreases as it follows the Bitmoji.
Once done, comment out this line using //
and save the script. You can later uncomment it to observe changes in the y position during jumps and slides.
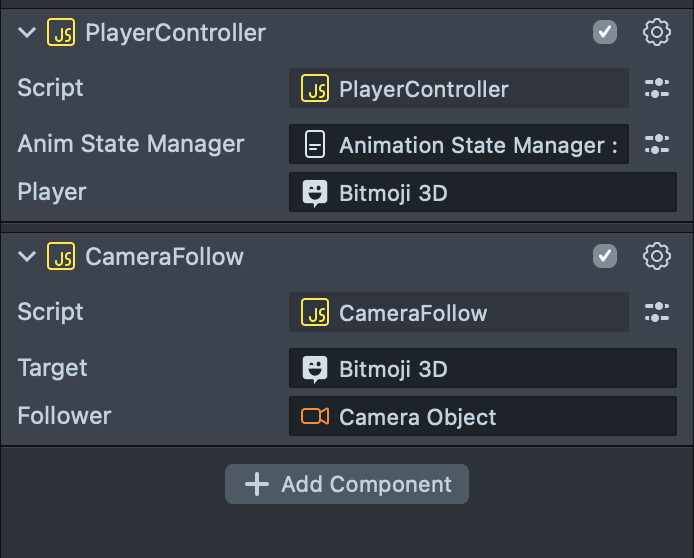
Implementing Jump Animation
In this section, we’ll enhance the Bitmoji’s movement by adding a jump animation triggered by a upSwipe
. The Bitmoji will move upward during the jump and smoothly return to the ground, seamlessly integrating with its running and side-to-side movements.
Start by importing the following animations from Mixamo or from here:
- Jump: We will use this animation to make the Bitmoji jump.
- Soccer Tackle: This will be used later for the slide movement.
Make sure to organize these animations by placing them in the Animations - Mixamo folder in your Asset Browser to keep your project tidy.
Next, we’ll add the Jump animation to the Animation Player of the Bitmoji.
Create a new clip in the Animation Player and assign the newly imported Jump asset to the Animation Asset.
Name the clip Jump and set the playback mode to Single, as we want the Bitmoji to jump only once each time it’s triggered.
Setting the playback mode to Single ensures that the animation plays through once without looping, perfectly aligning with a jump action.
In the Animation State Manager, create a new state for the jump animation.
Set the state name to Jump and choose Single Clip as the type.
Assign the previously created Jump clip to this state.
Then, add a new parameter in the Animation State Manager to control the Jump animation.
Name the parameter jump and set its type to Trigger.
This parameter will enable us to trigger the Jump animation later in response to a upSwipe
gesture.
Using a Trigger type ensures that the Jump animation is activated only once each time the user performs a swipe up gesture.
Unlike other parameter types, such as Bool or Float, which maintain their values until changed, a Trigger automatically resets itself after being activated.
Now, we will create two transitions in the Animation State Manager.
The first transition will go from the Specific State: RunBlendTree (the Bitmoji’s default running state) to the Specific State: Jump.
Set the transition duration to 0.20 and add a condition using the jump parameter we created earlier with the type Trigger.
This transition ensures that the Bitmoji moves seamlessly from running to jumping when the jump parameter is triggered.
A short transition time like 0.20 strikes a balance between responsiveness (immediate reaction to the jump trigger) and visual fluidity, making the transition feel natural and seamless.
The second transition will go from the Specific State: Jump back to the Specific State: RunBlendTree (the default running state).
Set the transition duration to 0.20 for a smooth return to the running state.
To signal when to exit the Jump state, set the Exit Time to 1.
By setting the Exit Time to 1, we are ensuring that the transition back to the RunBlendTree
state happens only after the Jump
animation has fully completed.
This guarantees that the Bitmoji finishes the entire jump sequence before returning to its default running state, making the transition feel complete and visually natural.
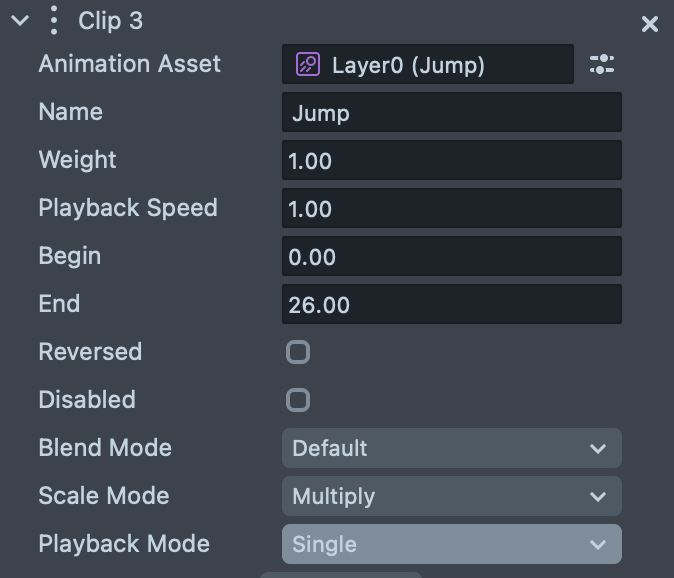
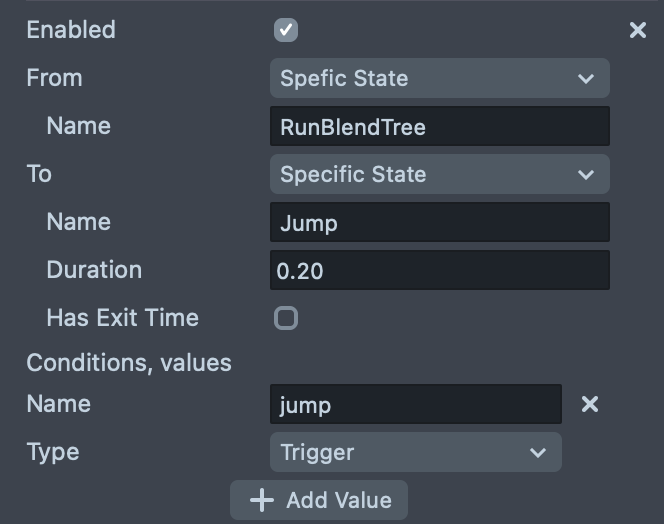
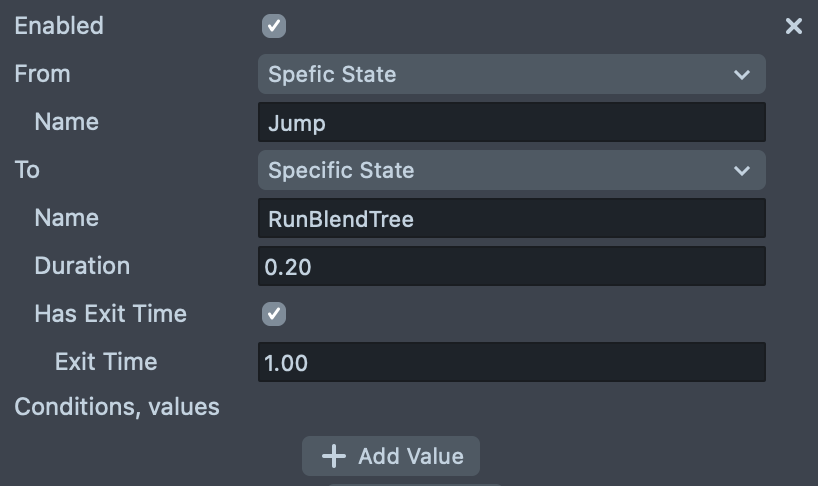
Implementing Jump Movement
Now that we’ve set up the animation settings for the Jump animation in the Animation State Manager, it’s time to bring it to life. Just like we triggered the left and right animations in the PlayerController script, we’ll implement the logic to trigger the Jump animation whenever the user performs a swipe up gesture.
Let’s start by opening the PlayerController script and navigating to the upSwipe
function.
In this function, we’ll use the setTrigger
method on the Animation State Manager
to activate the Jump animation when the user performs a swipe up gesture.
The setTrigger
method ensures that the Jump animation is triggered only when the swipe up gesture occurs, seamlessly integrating it with the existing animations.
After saving the script, let’s test it in the Preview Panel.
When we swipe up, the Bitmoji performs the Jump animation, which is great! But we noticed a couple of things:
- The jump isn’t high enough to clear obstacles.
- The distance covered during the jump is too small, which means it might not bypass obstacles effectively.
Enhancing both the height and distance of the jump will make the Bitmoji’s movements more practical for gameplay and allow it to navigate obstacles seamlessly.
To start, we need to know when the Bitmoji is in the Jump state so we can modify its y and z positions during the jump.
To achieve this, we’ll introduce a new jump
boolean variable.
When the user performs a swipe up gesture, we’ll set jump
to true
.
Then, in the onUpdate
function, we’ll check if jump
is true
to trigger the changes in the Bitmoji’s position.
To track how long the jump state has been active, we first need to know when it begins.
In the upSwipe
function, we’ll capture the exact time the jump starts using getTime()
and store it in the jumpStartTime
variable.
getTime()
: Returns the total time elapsed since the script started running.
This is useful for tracking specific events, like when the jump starts.
Now, in the onUpdate
function, after confirming that the Bitmoji is in the jump state, we need to calculate how long the jump has been in progress.
To do this, we will subtract the jumpStartTime
(the time when the jump started) from the current time using getTime()
.
Save the result in a variable called elapsedTime
.
This value will help us determine if the jump has been going on long enough to stop it or if it should continue.
By tracking the elapsedTime
, we ensure precise control over the jump’s duration and can create smooth transitions back to the running state when the jump is complete.
To control how long the Bitmoji stays in the jump state, we’ll introduce a jumpDuration
variable at the beginning of the script.
This variable will allow us to define the total duration of the jump.
Then, we’ll check if elapsedTime
is less than jumpDuration
to continue adjusting the y
and z
positions for the jump, which we will implement in the next step.
We’ll set the jumpDuration
to 1.5
seconds for now, but feel free to experiment with this value once the jump code is implemented to find the timing that feels right for your game.
To make the Bitmoji’s jump appear smooth and natural, we need to calculate the progress of the jump as a value between 0 and 1.
This will represent how far along the jump is at any given moment.
We calculate this by dividing elapsedTime
by jumpDuration
. The resulting jumpProgress
value will increase gradually from 0 (at the start of the jump) to 1 (at the end of the jump).
The jumpProgress
value helps us determine the current position of the Bitmoji along the jump's trajectory.
This ensures the jump height and motion are proportional to the time elapsed, creating a visually smooth animation.
To make the Bitmoji jump upwards and then come back down naturally, we’ll update the targetPos.y
value.
We use the Math.sin
function combined with jumpProgress
to create a smooth arc-like motion, mimicking a natural jump.
By multiplying jumpProgress
by Math.PI
, the sine function produces a curve that starts at 0, peaks at 1 (the highest point of the jump), and returns to 0 as the jump completes.
The value 30
represents the jump height. You can adjust this value to make the jump higher or lower.
Experiment with it to suit your desired gameplay.
Because the Bitmoji is already moving forward by decreasing the currentPos.z
value at the beginning of the onUpdate function, we’ll make it go even farther forward during the jump state.
By subtracting an additional value (e.g., 2
) from currentPos.z
, we create the effect of the Bitmoji leaping forward while jumping.
You can experiment with the value (e.g., 2
) to adjust how far the Bitmoji moves forward during the jump.
A higher value will make the jump feel more dynamic, while a lower value will make it more subtle.
If elapsedTime
is greater than or equal to jumpDuration
, we’ll stop the jump by resetting the jump<
variable to false
.
Finally, after the jump duration has elapsed, we need to reset the vertical position of the Bitmoji to bring it back to the ground.
Set targetPos.y = 0
to ensure the Bitmoji smoothly lands after the jump is complete.
Resetting targetPos.y
ensures the Bitmoji returns to its normal running position after completing the jump.
Now, save the script and test the jump in the Preview Panel.
Swipe up to trigger the jump animation and observe how the Bitmoji behaves.
Make sure it lands back to its normal running position seamlessly after completing the jump.
This is also the perfect time to experiment with different values for the jump duration, height, and forward distance to achieve the desired effect.
Try tweaking the jumpDuration
, the jump height, and the jump distance (the additional value subtracted from currentPos.z
) to customize the Bitmoji’s jump behavior.
Adjust these values to make the jump feel natural and suitable for your game design.
Implementing Slide Animation
In this section, we’ll enhance the Bitmoji’s movements further by adding a slide animation triggered by a downSwipe
gesture. The Bitmoji will slide forward, making it easier to pass under obstacles seamlessly.
To implement the Slide animation, we’ll follow a similar approach to the Jump animation. Before proceeding, try implementing the Slide animation steps on your own based on what you learned in the Jump animation section. Once done, compare your work with the steps below to ensure everything is set up correctly.
Start by adding the Soccer Tackle animation to the Animation Player of the Bitmoji.
Create a new clip and assign the imported Soccer Tackle asset to the Animation Asset.
Name the clip Slide and set the playback mode to Single, as the sliding action should play only once when triggered.
Setting the playback mode to Single ensures that the slide animation doesn’t repeat, perfectly aligning with a quick slide action.
In the Animation State Manager, create a new state for the slide animation.
Set the state name to Slide and choose Single Clip as the type.
Assign the previously created Slide clip to this state.
Add a new parameter in the Animation State Manager to control the Slide animation.
Name the parameter slide and set its type to Trigger.
This parameter will allow us to trigger the Slide animation later in response to a downSwipe
gesture.
Using a Trigger type ensures the Slide animation activates only when required, preventing accidental repetitions.
Now, create a transition in the Animation State Manager to go from the Specific State: RunBlendTree to the Specific State: Slide.
Set the transition duration to 0.20 and add a condition using the slide parameter with the type Trigger.
This transition ensures that the Bitmoji moves smoothly from running to sliding when the Slide parameter is triggered, making the motion feel fluid and responsive.
Next, create a second transition to return from the Specific State: Slide to the Specific State: RunBlendTree.
Set the transition duration to 0.20 and enable Has Exit Time with an Exit Time of 1 to ensure the transition happens after the slide animation completes.
Enabling Has Exit Time with a value of 1 ensures the Slide animation finishes completely before returning to the running state, maintaining smoothness and visual consistency.
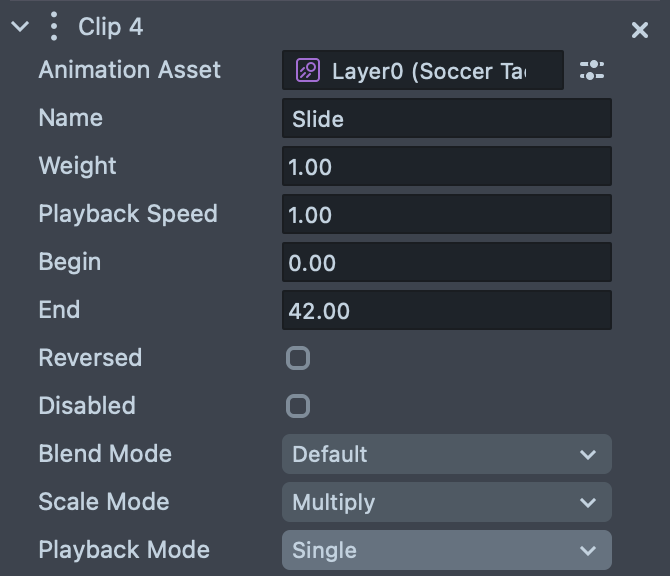
Implementing Slide Movement
With the animation settings for the Slide action in place, it’s time to make the Bitmoji perform the sliding motion. Similar to the jump, we’ll trigger the Slide animation in the PlayerController script when the user performs a downSwipe
gesture.
Before proceeding, try implementing the Slide movement steps on your own based on what you learned in the Jump movement section. Once done, compare your work with the steps below to ensure everything is working as intended.
Let’s start by opening the PlayerController script and navigating to the downSwipe
function.
In this function, we’ll use the setTrigger
method on the Animation State Manager
to activate the Slide animation.
The setTrigger
method ensures that the Slide animation is triggered only once when the user performs a downSwipe
gesture.
Now, save the script and test the sliding behavior in the Preview Panel by swiping down.
Observe that the Bitmoji plays the Slide animation when triggered.
However, to ensure it passes successfully under obstacles, we need to adjust its movement during the slide by decreasing the z
position more than when it is running.
As we did for the jump, we’ll further decrease the z
position while the Bitmoji is in the sliding state to make the movement functional for obstacle avoidance.
In the downSwipe function, we’ll introduce a slide
boolean variable and set it to true
.
We’ll also store the start time of the slide in a variable called slideStartTime
using getTime()
. This helps us track how long the slide state has been active.
The slide
variable will allow us to manage the slide state in the onUpdate function later, while the slideStartTime
ensures we can measure the slide duration accurately.
Next, in the onUpdate function, we’ll check if the slide
variable is true
.
If it is, calculate how long the Bitmoji has been sliding by subtracting the slideStartTime
from the current time using getTime()
.
Save this value in an elapsedTime
variable.
Tracking the elapsedTime
ensures we have precise control over the slide duration, stopping it at the right moment.
To determine how long the Bitmoji stays in the slide state, we’ll introduce a slideDuration
variable at the beginning of the script.
In the onUpdate
function, we’ll check if the time spent sliding (tracked by elapsedTime
) is still less than slideDuration
. If it is, we’ll decrease the currentPos.z
value by 2
to make the Bitmoji slide forward.
We’ve moved the Bitmoji slightly forward by decreasing currentPos.z
by 2
.
You can experiment with this value, along with the slideDuration
, to adjust how far and how fast the Bitmoji slides to better suit your game.
Finally, if elapsedTime
exceeds the slideDuration
, we’ll reset the slide
variable to false
.
This ensures that the sliding action stops and the Bitmoji returns to its default running behavior.
Resetting the slide
variable ensures the Bitmoji transitions back to its normal state after sliding.
Save the script and test it in the Preview Panel.
Swipe down to trigger the Slide animation and observe how the Bitmoji slides forward. Experiment with the slideDuration
and slideDistance
values to achieve the desired effect.
Tuning the slideDuration
and slideDistance
will help you create a slide that feels smooth and practical for your game.
Final Adjustments and Best Practices
Fine-Tuning Movement and Keeping Your Project Organized
Now that the forward, jump and slide movements are implemented, you can experiment with all the values we’ve introduced to achieve the desired gameplay feel.
For example:
- Adjust the
jumpHeight
to make the jump higher or lower. - Modify the
jumpDuration
to control how long the Bitmoji stays in the air. - Fine-tune the
slideDuration
and thez
decrement value to customize the slide speed and distance.
Tweaking these values will help you refine the player’s movements, making the gameplay smoother and more enjoyable.
Ensure all variables are declared at the beginning of your script before using them.
This includes constants like slideDuration
, jumpDuration
, and variables like jump
or slideStartTime
.
Declaring variables at the top of your script improves code readability and prevents errors from undefined variables.
Let’s take this a step further and create variables for all other hardcoded values in the script, such as jumpHeight
(currently set to 30) and jumpForwardOffset
and slideForwardOffset
.
By defining these as variables, you can easily adjust them later without needing to search through your code, making it more modular and easier to maintain.
Replacing hardcoded values with variables improves flexibility, allowing you to experiment with different gameplay settings and make quick changes without introducing errors.
Keep your project organized as it grows:
- Place all new animation files, such as Jump and Slide, in the Animations - Mixamo folder.
- Ensure your scripts are neatly stored in a Scripts folder for easy access.
Organizing assets and scripts now will save you time as your project becomes larger and more complex.
Finally, test your project in the Preview Panel by performing all actions:
- Swipe left and right to confirm side-to-side movement.
- Swipe up to test the jump height and forward movement.
- Swipe down to verify the slide distance and speed.
Thorough testing ensures everything works together seamlessly, providing a polished user experience.
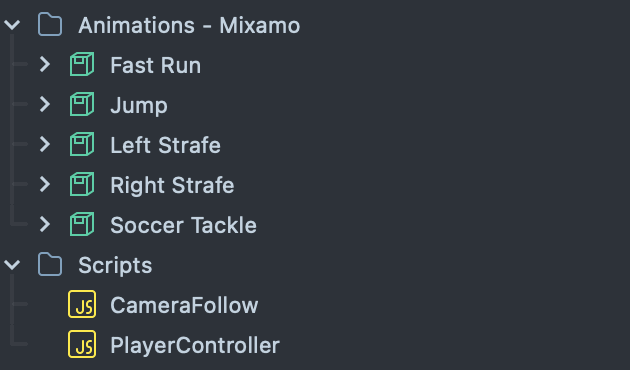
Summary
In this part of the Bitmoji Runner Game tutorial, you've expanded the functionality of your game by implementing forward movement, jump, and slide mechanics for your Bitmoji character. You learned how to smoothly move the player along the z-axis while ensuring the camera follows it, keeping the character in view. Additionally, you integrated new animations and implemented logic to trigger the jump and slide movements based on swipe gestures.
By fine-tuning parameters like jump height, jump duration, and slide speed, you now have greater control over the gameplay experience.
Tip: Experiment with the values you’ve created to achieve the perfect feel for the jump and slide mechanics. Keep your project organized by maintaining a clear structure for scripts and assets as you prepare for more advanced features in the next part!